Auto-reply function for Email Inbox
Rossum's extension environment enables you to customize various day-to-day workflows. One such workflow is to automatically reply to emails sent to Rossum's smart inbox. Automatic replies can be sent when receiving emails with no attachments or containing specific content. You can set it up very quickly directly in the UI. You can read more about it here.
Setting up auto-reply function
Your auto-reply logic could look as follows:
- Filter the list of received attachments in one email
- Use Rossum's emailing API to reply if no suitable attachment is found automatically
To achieve such behavior, create a custom function and assign the function to the "email.received" event action. Afterward, use the code below to reach the desired behavior.
from urllib import request, parse
from urllib.error import HTTPError
import json
import re
"""
The rossum_hook_request_handler is an obligatory main function that accepts
input and produces output of the rossum custom function hook.
:param payload: see https://api.elis.rossum.ai/docs/#annotation-content-event-data-format
:return: dict with files to be processed
"""
def rossum_hook_request_handler(payload):
if payload['event'] == 'email' and payload["action"] == "received":
try:
files = main(payload)
except Exception as e:
return payload["files"]
return {"files": files}
"""
Main function describes the auto-reply feature.
:param payload: dict representing the payload
:return: dict with the API response
"""
def main(payload):
incoming_files = payload["files"]
auth_token = payload["rossum_authorization_token"]
if len(incoming_files) == 0:
if "headers" in payload:
#subject = "Auto-reply for: {0}".format(payload["headers"]["subject"])
subject = "Ignored email: {0}".format(payload["headers"]["subject"])
from_text = payload["headers"]["from"]
email = parse_email(from_text)
#date = payload["headers"]["date"]
#content = "Dear sender,\n\nwe have received an email with no attachments on {0}.\n\nBest regards".format(date)
content = "Dear sender,\n\nwe have just received an email with no recognized attachments.\n\nOnly documents attached as PDF (or JPEG, PNG or TIFF) files are accepted.\n\n-- \n"
response = send_email(subject, email, content, auth_token)
return incoming_files
"""
Use the Rossum email API to send email notifications.
:param subject: subject of the email
:param email: email of the recipient
:param message: message to be sent
:param auth_token: auth token for accessing the Rossum API
:return: None
"""
def send_email(subject, email, message, auth_token):
payload = {
#"to": [{"email": email, "name": "Rossum Intelligent Inbox"}],
"to": [{"email": email}],
"template_values":{
"subject": subject,
"message": message
}
}
data = json.dumps(payload)
data = data.encode("utf-8")
req = request.Request("https://example.rossum.app/api/v1/emails/send", data=data)
req.add_header('Authorization', "token {0}".format(auth_token))
req.add_header('Content-Type', "application/json")
try:
r = request.urlopen(req)
return r.getcode()
except HTTPError as x:
return {}
return {}
"""
Parse email from the "from" text
:param from_text: "from" representing the name and email of the sender
:return: string - parsed email
"""
def parse_email(from_text):
match = re.search(r'[\w\.-]+@[\w\.-]+', from_text)
email = match.group(0)
return email
When setting up the auto-reply custom function, you should:
-
Ensure your organization can use custom functions that can access the internet. If you need more clarification, please ask us at [email protected].
-
Attribute token_owner of your hook should be set up so that you can authenticate to Rossum's API with an authentication token. It can now be set in the Rossum's extension detail, as shown in the image below.
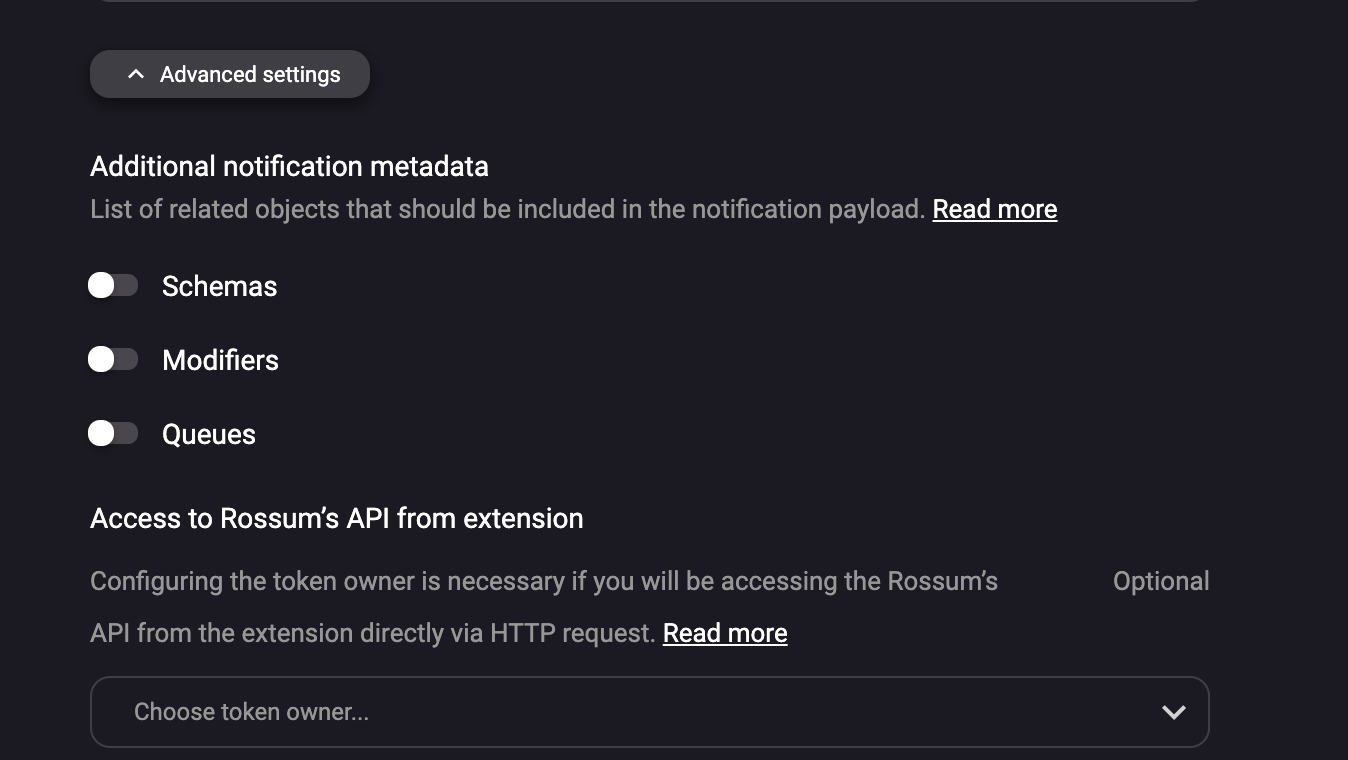
Enabling access to the Rossum API from extension.
Updated 6 months ago