Two-way PO Matching With Custom Function
Two-way matching (matching of an invoice to a purchase order) is an essential process in AP automation. Rossum can already capture the data from invoice so why not make it pair captured data from the invoice with the purchase order (PO) master data.
In this article you will learn how to:
- Upload purchase order master data to Rossum's Data matching extension/database
- Implement a custom function that performs the two way matching
Storing PO master data in Rossum
You can upload your purchase order data into Rossum over the Data matching extension UI. You can take for example the following JSON file, upload it in the UI, select po_number as a unique identifier of the uploaded records and confirm the upload.
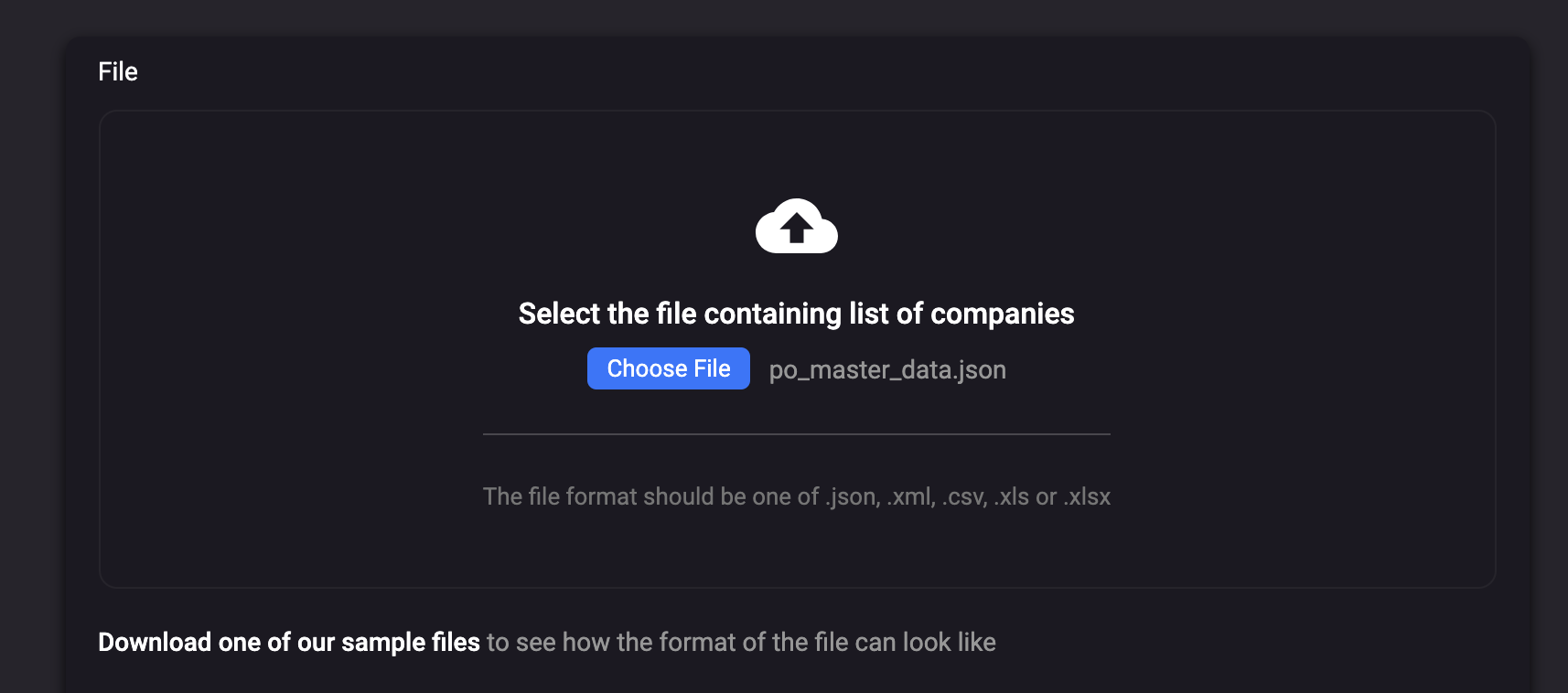
Uploading file with PO master data.
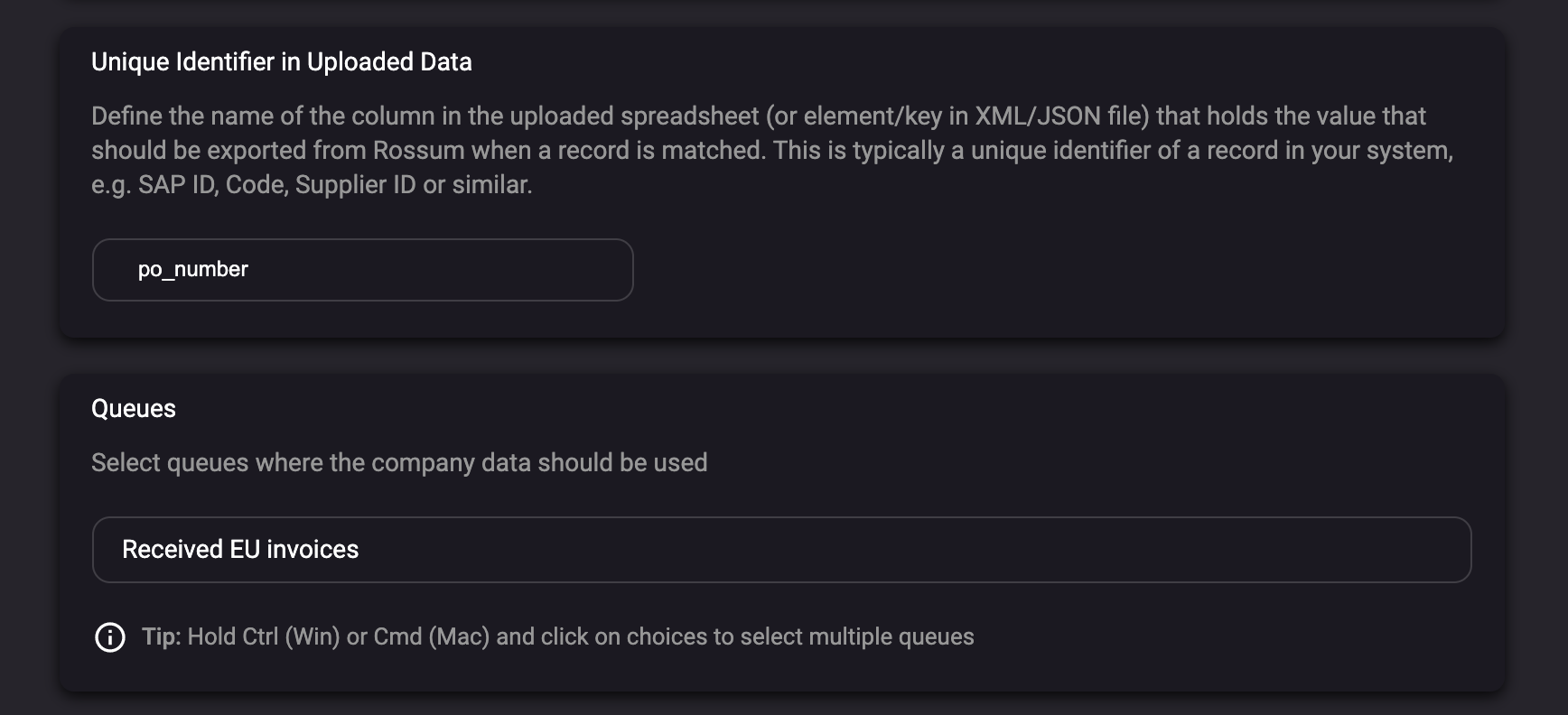
Selecting the unique identifier in the master data.
Example of the uploaded JSON file.
[
{
"po_number": "1",
"total_amount": 2520,
"line_items": [
{
"item_sku": "123",
"item_description": "Creme liquide semi epaisse",
"item_quantity": 50,
"item_amount_base": 10,
"item_amount_total": 500
},
{
"item_sku": "124",
"item_description": "Beurre Demi-sel Moule de Bretagne",
"item_quantity": 60,
"item_amount_base": 20,
"item_amount_total": 1200
},
{
"item_sku": "125",
"item_description": "Beurre Doux Tendre",
"item_quantity": 40,
"item_amount_base": 10,
"item_amount_total": 400
}
]
},
{
"po_number": "2",
"total_amount": 420,
"line_items": [
{
"item_sku": "126",
"item_description": "Lait Demi-Ecreme Bio",
"item_quantity": 35,
"item_amount_base": 10,
"item_amount_total": 350
}
]
},
{
"po_number": "3",
"total_amount": 480,
"line_items": [
{
"item_sku": "127",
"item_description": "Lait Demi-Ecreme",
"item_quantity": 400,
"item_amount_base": 10,
"item_amount_total": 400
}
]
}
]
If you would like to upload the data periodically over the API, you can use the /import API endpoint.
Using a custom function for two-way matching
In order to accomplish a real two-way matching behavior, you should:
- Capture the PO number and line items on the invoice
- Lookup the purchase order in the master database.
- Try to match the line items from invoice to line items in the PO record.
- Automate the document if possible.
Thanks to a combination of Rossum's custom function and the Data matching master data, this logic is very easy to replicate in Rossum.
Below you can explore a custom function that:
- Maps fields from Extraction schema to uploaded master data
- Defines strategies for matching the line items (exact/fuzzy/tolerance based matching)
- Retrieves the captured PO number if present
- Fetches the PO number data from Data matching master database over the HTTP API.
- Implements the matching logic of line items
- Shows custom user messages if matching not successful or automates the fields.
The PO matching function expects that you:
- have field with schema ID po_number in the extraction schema
- have line items setup in the extraction schema with "line_item" ID
"""
Line items PO matching extension
"""
# Mapping of the extraction schema IDs to Master Data column IDs
mapping_schema_to_master_data = {
"item_code": "item_sku",
"item_description": "item_description",
"item_quantity": "item_quantity",
"item_amount_base": "item_amount_base",
"item_amount_total": "item_amount_total",
"po_number": "po_number",
}
# Multiple ways how to match items
# N.B. fields are matched in the specified order. The first field is considered
# as the primary key, which influences how mismatches are reported to the user.
matching_strategies = [
[
{"id": "item_code", "method": "exact"},
{"id": "item_quantity", "method": "exact"},
{"id": "item_amount_total", "method": "tolerance", "threshold": 0.1, "max_limit": 30,},
],
[
{"id": "item_description", "method": "fuzzy", "threshold": 0.7},
{"id": "item_quantity", "method": "exact"},
{"id": "item_amount_total", "method": "tolerance", "threshold": 0.1, "max_limit": 30,},
],
]
DATA_MATCHING_FIELD = "po_number" # Extraction schema field to be used for matching.
DATA_MATCHING_BUTTON = "master_data_match_po" # Button for validating items.
SCHEMA_ITEMS_ID = "line_item" # Field representing line items in extracted data
MASTER_ITEMS_ID = "line_items" # Field representing line itens in Master Data
#################################################################
# Configuration section ends with this line
#################################################################
from collections import defaultdict
from difflib import SequenceMatcher
import json
from urllib import request, parse
from urllib.error import HTTPError
HTTP_COOKIE = None
def rossum_hook_request_handler(payload):
"""
The rossum_hook_request_handler is an obligatory main function that accepts
input and produces output of the rossum custom function hook.
:param payload: see https://api.elis.rossum.ai/docs/#annotation-content-event-data-format
:return: messages and operations that update the annotation content or show messages
"""
if payload["event"] == "annotation_content" and (
payload["action"] == "user_update" or payload["action"] == "initialize"
):
messages = []
operations = []
try:
messages, operations = main(payload)
except Exception as e:
messages = [create_message("error", "Serverless Function: " + str(e))]
return {"messages": messages}
return {"messages": messages, "operations": operations}
def main(payload):
"""
Main function describes the desired data matching behavior of the function.
:param payload: queue ID where the master data is stored.
:param value: lookup value
:param master_field_id: ID of the column/field which should be used for lookup
:return: dict with the API response
"""
updated_datapoints = payload["updated_datapoints"]
content = payload["annotation"]["content"]
# The custom logic should trigger only when:
# 1. Data is initially extracted. (initialize event && no updated_datapoints)
# 2. Annotation is opened in validation screen (user_update event && no updated_datapoints)
# 3. DATA_MATCHING_FIELD is updated in validation screen (user_update && updated field)
# 4. DATA_MATCHING_BUTTON is pressed on user validation screen (user_update && triggered button)
if payload["action"] == "user_update" and len(updated_datapoints) > 0:
updated_field = find_by_datapoint_id(content, updated_datapoints[0])
if (
updated_field
and updated_field["schema_id"] != DATA_MATCHING_FIELD
and updated_field["schema_id"] != DATA_MATCHING_BUTTON
):
return [], []
# Login to Rossum's Data Matching API
auth_token = payload["rossum_authorization_token"]
response = login_with_token(auth_token)
if response is None:
return (
[create_message("error", "Could not login to Rossum's Data matching API", None)],
[],
)
captured_items = find_by_schema_id(content, SCHEMA_ITEMS_ID)
matching_field = find_by_schema_id(content, DATA_MATCHING_FIELD)[0]
matching_field_value = matching_field["content"]["value"]
queue_id = payload["annotation"]["queue"].split("/")[-1]
response = lookup_item_in_master_data(
queue_id=queue_id, lookup_value=matching_field_value, master_field_id=DATA_MATCHING_FIELD,
)
if response is None:
return (
[
create_message(
"error", "Problem with the search endpoint of Rossum's Data matching API", None,
)
],
[],
)
elif "matches" in response and len(response["matches"]) == 0:
return (
[create_message("warning", "Could not find PO match", matching_field["id"])],
[],
)
first_match = response["matches"][0]
master_items = find_items_in_master_data(first_match, MASTER_ITEMS_ID)
if master_items is None:
return (
[create_message("warning", "Could not find any items in the matched record")],
[],
)
messages, operations = match_captured_items_to_master_items(
captured_items, master_items, matching_field
)
return messages, operations
def login_with_token(token):
"""
Login to the Data Matching API. User has to be logged in when using other endpoints.
:param token: auth token provided by the Rossum API /login endpoint - https://api.elis.rossum.ai/docs/#login
:return: dict with the API response
"""
payload = {}
data = parse.urlencode(payload).encode("ascii")
req = request.Request("https://data-matching.elis.rossum.ai/api/v1/auth/token_login", data=data)
req.add_header("Authorization", "Token {0}".format(token))
try:
with request.urlopen(req) as resp:
response = json.loads(resp.read().decode("utf-8"))
global HTTP_COOKIE
HTTP_COOKIE = resp.headers.get("Set-Cookie")
except HTTPError:
return None
return response
def lookup_item_in_master_data(queue_id, lookup_value, master_field_id):
"""
Search the Data matching database over the API. - https://data-matching.elis.develop.r8.lol/api/v1/documentation -> /match/search
:param queue_id: queue ID where the master data is stored.
:param lookup_value: lookup value
:param master_field_id: ID of the column/field which should be used for lookup
:return: dict with the API response
"""
payload = {
"queue_id": queue_id,
"extracted_value": lookup_value,
"fuzzy": False,
"compound_match": False,
"joining_char": ", ",
"matching_keys": [master_field_id],
}
data = json.dumps(payload)
data = data.encode("utf-8")
req = request.Request("https://data-matching.elis.rossum.ai/api/v1/match/search", data=data)
req.add_header("Cookie", HTTP_COOKIE)
req.add_header("Content-Type", "application/json")
try:
with request.urlopen(req) as resp:
response = json.loads(resp.read().decode("utf-8"))
except HTTPError:
return None
return response
def find_items_in_master_data(content, master_field_id):
"""
Find a specific field in the response from the /search endpoint.
:param content: dict representing the matched item
:param master_field_id: ID of the field to be found in the matched record.
:return: dict representin the matched field
"""
for match in content["related_data"]:
if match["matching_key"] == master_field_id:
return json.loads(match["value"])
return None
def match_captured_items_to_master_items(captured_items, master_items, matching_field):
"""
Take captured items and master data items and match them together.
Various matching strategies will be used for matching.
:param captured_items: list of captured items
:param master_items: list of master data items
:return: messages and operations to be returned to the Rossum app
"""
operations = []
messages = []
# Try one matching rule for all lines. If not successful, try next one.
for matching_strategy in matching_strategies:
(
all_items_matched,
new_operations,
new_messages,
) = match_captured_items_to_master_items_by_specific_rule(
captured_items, master_items, matching_strategy
)
if all_items_matched:
messages.append(
create_message(
message_type="info",
message_content="All lines were matched to PO",
datapoint_id=None,
)
)
operations.append(
create_automation_operation(matching_field, automation_type="connector")
)
# At least some item was matched => show automation + user messages
if len(new_operations) > 0:
messages.extend(new_messages)
operations.extend(new_operations)
break
if len(operations) == 0:
messages.append(
create_message(
message_type="info",
message_content="No items were matched to PO",
datapoint_id=None,
)
)
return messages, operations
def match_captured_items_to_master_items_by_specific_rule(
captured_items, master_items, matching_strategy
):
"""
Use a specific matching strategy for matching captured items and master items.
:param captured_items: list of captured items
:param master_items: list of master data items
:param matching_strategy: a specific matching strategy to be used
:return: tuple with (bool saying if all items were matched, operations, messages)
"""
operations = []
messages = []
all_items_matched = True
master_items_indexes = [i for i in range(0, len(master_items))]
for captured_item in captured_items:
(matched_line_item, new_operations, new_messages,) = match_captured_item_to_master_items(
captured_item, master_items_indexes, master_items, matching_strategy
)
if not matched_line_item:
all_items_matched = False
operations.extend(new_operations)
messages.extend(new_messages)
return all_items_matched, operations, messages
def match_captured_item_to_master_items(
captured_item, master_items_indexes, master_items, matching_strategy
):
"""
Try to match a single captured item to master data items
:param captured_items: captured item
:param master_items_indexes: list of master data indexes that were not yet matched
:param master_items: list of master data items
:param matching_strategy: specific matching strategy to be used
:return: tuple with (bool saying the item was matched, operations, messages)
"""
primary_key_mismatch = None
# Map of cell datapoint ids to a list of mismatches (only for cases where
# primary key matched)
other_field_mismatches = defaultdict(list)
for master_item_index in master_items_indexes:
master_item = master_items[master_item_index]
matched_field_ids, mismatched_fields = match_master_item(
master_item, captured_item, matching_strategy
)
if not mismatched_fields:
# Remove the matched index since it was already matched
master_items_indexes.remove(master_item_index)
# Enable automation of all fields, not just matched_field_ids
operations = [
create_automation_operation(item, automation_type="connector")
for item in captured_item["children"]
]
return True, operations, []
elif mismatched_fields:
if mismatched_fields[0][1] is None:
primary_key_mismatch = mismatched_fields[0][0]
else:
for mismatched_field, mismatched_value in mismatched_fields:
other_field_mismatches[mismatched_field["id"]].append(mismatched_value)
messages = []
if other_field_mismatches:
# At least one master data row matched the primary key, report details
for datapoint_id, values in other_field_mismatches.items():
if len(values) == 1:
message_content = f'Must be "{values[0]}" to match the PO line'
else:
message_content = f"Must be one of {', '.join(values)} to match the PO line"
messages.append(
create_message(
message_type="warning",
message_content=message_content,
datapoint_id=datapoint_id,
)
)
elif primary_key_mismatch:
messages.append(
create_message(
message_type="warning",
message_content="Could not match to any PO line",
datapoint_id=primary_key_mismatch["id"],
)
)
return False, [], messages
def match_master_item(master_item, captured_item, matching_strategy):
"""
Try to match a single captured item to master item by a specific strategy
:param master_item: dict representing the master data item
:param captured_item: dict representing the captured item
:param matching_strategy: specific matching strategy to be used
:return: tuple of (matched_field_ids, mismatched_fields)
matched_field_ids is a list of schema ids of successfuly matched fields.
mismatched_fields is a list of tuples (captured_datapoint, master_value).
"""
matched_field_ids = []
mismatched_fields = []
for field_strategy in matching_strategy:
if field_strategy["id"] not in mapping_schema_to_master_data:
continue
master_item_value = master_item[mapping_schema_to_master_data[field_strategy["id"]]]
captured_item_datapoint = find_field_in_item(captured_item, field_strategy["id"])
if master_item_value == None or captured_item_datapoint == None:
continue
captured_item_value = (
None
if captured_item_datapoint["content"]["normalized_value"] == "None"
else captured_item_datapoint["content"]["normalized_value"]
)
if captured_item_value is not None:
captured_item_value = float(captured_item_value)
else:
captured_item_value = captured_item_datapoint["content"]["value"]
field_matched = match_field(
captured_item_value, captured_item_datapoint, master_item_value, field_strategy,
)
if field_matched:
matched_field_ids.append(field_strategy["id"])
elif not matched_field_ids:
# Primary key mismatch means no further matching necessary
return [], [(captured_item_datapoint, None)]
else:
# If the first key did match, collect all remaining mismatches as well
# as reference values
mismatched_fields.append((captured_item_datapoint, master_item_value))
return matched_field_ids, mismatched_fields
def match_field(captured_item_value, captured_item_datapoint, master_item_value, matching_strategy):
"""
Try to match a specific captured field and specific master data field
:param captured_item_datapoint: dict representing a captured field
:param master_item_value: value of the master data field
:param matching_strategy: specific matching strategy to be used
:return: bool saying whether the field was matched
"""
if isinstance(captured_item_value, str) and matching_strategy["method"] == "fuzzy":
# Fuzzy matching on strings
similarity = compute_similarity(captured_item_value, master_item_value)
if similarity >= matching_strategy["threshold"]:
return True
elif isinstance(captured_item_value, float) and matching_strategy["method"] == "tolerance":
# Numbers can differ slightly
difference = abs(captured_item_value - master_item_value)
if (
difference <= matching_strategy["max_limit"]
and (difference / master_item_value) < matching_strategy["threshold"]
):
return True
else:
if master_item_value == captured_item_value:
return True
return False
def find_field_in_item(item, schema_id):
"""
Search for a specific field in a list of fields.
:param item: list of dicts representing fields
:param schema_id: string representing a specific field
:return: dict representing the field
"""
for field in item["children"]:
if field["schema_id"] == schema_id:
return field
return None
def find_by_schema_id(content, schema_id: str):
"""
Return datapoints matching a schema id.
:param content: annotation content tree (see https://api.elis.rossum.ai/docs/#annotation-data)
:param schema_id: field's ID as defined in the extraction schema(see https://api.elis.rossum.ai/docs/#document-schema)
:param accumulator: list for accumulating values with the same schema_id (f.e. values from same table column)
:return: the list of datapoints matching the schema ID
"""
accumulator = []
for node in content:
if node["schema_id"] == schema_id:
accumulator.append(node)
elif "children" in node:
accumulator.extend(find_by_schema_id(node["children"], schema_id))
return accumulator
def find_by_datapoint_id(content, datapoint_id):
"""
Find specific datapoint by a datapoint ID.
:param content: annotation content tree (see https://api.elis.rossum.ai/docs/#annotation-data)
:param datapoint_id: ID of a specific datapoint
:return: dict representing the found field
"""
for node in content:
if node["id"] == datapoint_id:
return node
elif "children" in node:
result = find_by_datapoint_id(node["children"], datapoint_id)
if result:
return result
else:
continue
return None
def create_message(message_type, message_content, datapoint_id=None):
"""
Create a message which will be shown to the user
:param message_type: type of the message, any of {info|warning|error}. Errors prevent confirmation in the UI.
:param message_content: message shown to the user
:param datapoint_id: id of the datapoint where the message will appear (None for "global" messages).
:return: dict with the message definition (see https://api.elis.rossum.ai/docs/#annotation-content-event-response-format)
"""
return {
"content": message_content,
"type": message_type,
"id": datapoint_id,
}
def create_automation_operation(datapoint, automation_type):
"""
Enable automation of a specific field by updating its validation sources.
:param datapoint: content of the datapoint
:param automation_type: type of the automation validation source to be used
:return: dict with replace operation definition (see https://api.elis.rossum.ai/docs/#annotation-content-event-response-format)
"""
validation_sources = datapoint["validation_sources"]
if automation_type not in validation_sources:
validation_sources.append(automation_type)
return {
"op": "replace",
"id": datapoint["id"],
"value": {"validation_sources": validation_sources},
}
def compute_similarity(a, b):
"""
Compute similarity of two string values.
:param a: first string value
:param b: second string value
:return: float - representing the similarity
"""
return SequenceMatcher(None, a, b).ratio()
When setting up the PO matching custom function, you should:
- Make sure your organization is allowed to use custom functions that can access the internet. If you're not sure, please ask us at [email protected].
- Attribute token_owner of your hook should be setup so that you could authenticate to the Rossum's API with an authentication token. This can now be set in the Rossum's UI, as shown on the image below.
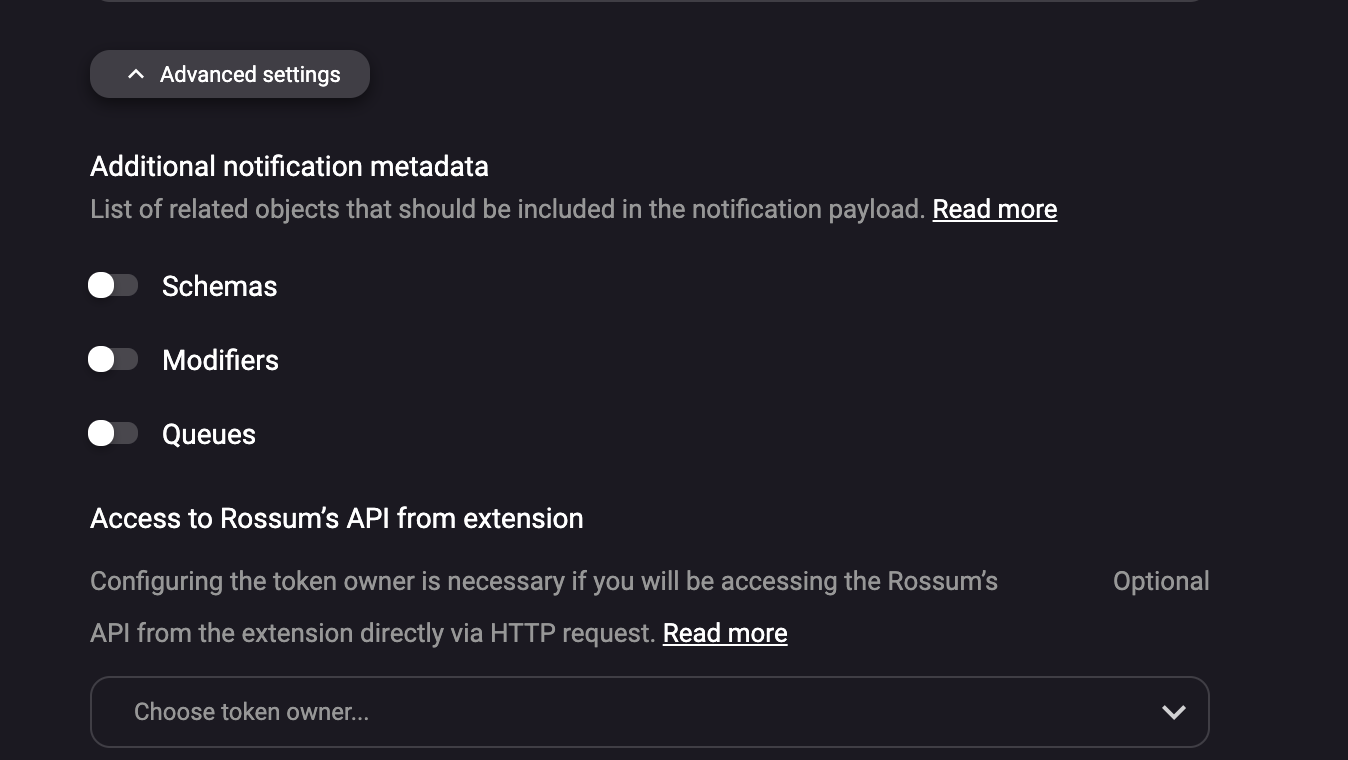
Enabling access of the extension to the Rossum's API.
Example of the Extraction schema to be used
Below is the example of the extraction schema to be used.
[
{
"category": "section",
"id": "invoice_info_section",
"label": "Basic information",
"hidden": false,
"children": [
{
"width_chars": 10,
"rir_field_names": [
"document_id",
"var_sym"
],
"constraints": {
"required": false
},
"score_threshold": 0.8,
"default_value": null,
"category": "datapoint",
"id": "invoice_id",
"label": "Invoice number",
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [
"order_id",
"sender_order_id"
],
"constraints": {
"required": true
},
"default_value": null,
"category": "datapoint",
"id": "po_number",
"label": "Order number",
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [
"date_issue"
],
"constraints": {
"required": false
},
"score_threshold": 0.8,
"default_value": null,
"category": "datapoint",
"id": "date_issue",
"label": "Issue date",
"type": "date",
"format": "D.M.YYYY"
},
{
"width_chars": 10,
"rir_field_names": [
"date_due"
],
"constraints": {
"required": false
},
"score_threshold": 0.8,
"default_value": null,
"category": "datapoint",
"id": "date_due",
"label": "Due date",
"type": "date",
"format": "D.M.YYYY"
},
{
"width_chars": 10,
"rir_field_names": [
"date_uzp"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "date_uzp",
"label": "Tax point date",
"hidden": true,
"type": "date",
"format": "D.M.YYYY"
},
{
"width_chars": 10,
"rir_field_names": [],
"constraints": {
"required": false
},
"default_value": "Lacté",
"category": "datapoint",
"id": "sender_name",
"label": "Sender name",
"hidden": true,
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [],
"constraints": {
"required": false
},
"default_value": "Dairy Products Ltd.",
"category": "datapoint",
"id": "recipient_name",
"label": "Recipient name",
"hidden": true,
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [
"email_header:from"
],
"constraints": {
"required": false
},
"default_value": "[email protected]",
"category": "datapoint",
"id": "email",
"label": "Email",
"hidden": true,
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [],
"constraints": {
"required": false
},
"default_value": "",
"category": "datapoint",
"id": "erp_status",
"label": "ERP status",
"hidden": true,
"type": "string"
}
],
"icon": null
},
{
"category": "section",
"id": "payment_info_section",
"label": "Payment instructions",
"hidden": true,
"children": [
{
"width_chars": 10,
"rir_field_names": [
"var_sym"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "var_sym",
"label": "Payment reference",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"account_num"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "account_num",
"label": "Account number",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"bank_num"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "bank_num",
"label": "Bank code",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"iban"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "iban",
"label": "IBAN",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"bic"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "bic",
"label": "BIC/SWIFT",
"hidden": true,
"type": "string",
"can_export": false
}
],
"icon": null
},
{
"category": "section",
"id": "amounts_section",
"label": "VAT & Amounts",
"hidden": false,
"children": [
{
"width_chars": 10,
"rir_field_names": [
"amount_total_base"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "amount_total_base",
"label": "Total without tax",
"hidden": true,
"type": "number",
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"amount_total_tax"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "amount_total_tax",
"label": "Total tax",
"hidden": true,
"type": "number",
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"amount_total"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "amount_total",
"label": "Amount total",
"type": "number",
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"currency"
],
"constraints": {
"required": false
},
"default_value": "EUR",
"category": "datapoint",
"id": "currency",
"label": "Currency",
"hidden": true,
"type": "string"
},
{
"category": "multivalue",
"id": "tax_details",
"label": "VAT Rates",
"hidden": true,
"children": {
"category": "tuple",
"id": "tax_detail",
"label": "VAT Rates",
"hidden": true,
"children": [
{
"width_chars": 10,
"rir_field_names": [
"tax_detail_rate"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "tax_detail_rate",
"label": "VAT Rate",
"hidden": true,
"type": "number",
"can_export": false,
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"tax_detail_base"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "tax_detail_base",
"label": "VAT Base",
"hidden": true,
"type": "number",
"can_export": false,
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"tax_detail_tax"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "tax_detail_tax",
"label": "VAT Amount",
"hidden": true,
"type": "number",
"can_export": false,
"format": "# ##0.#"
}
],
"rir_field_names": [
"tax_details"
]
},
"min_occurrences": null,
"max_occurrences": 4,
"default_value": null,
"show_grid_by_default": false,
"rir_field_names": null
}
],
"icon": null
},
{
"category": "section",
"id": "vendor_section",
"label": "Vendor & Customer",
"hidden": true,
"children": [
{
"width_chars": 10,
"rir_field_names": [
"sender_ic"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "sender_ic",
"label": "Vendor company ID",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"sender_vat_id",
"sender_dic"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "sender_vat_id",
"label": "Vendor VAT number",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"recipient_ic"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "recipient_ic",
"label": "Customer company ID",
"hidden": true,
"type": "string",
"can_export": false
},
{
"width_chars": 10,
"rir_field_names": [
"recipient_vat_id",
"recipient_dic"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "recipient_vat_id",
"label": "Customer VAT number",
"hidden": true,
"type": "string",
"can_export": false
}
],
"icon": null
},
{
"category": "section",
"id": "line_items_section",
"label": "Line items",
"hidden": false,
"children": [
{
"category": "multivalue",
"id": "line_items",
"label": "Line item",
"hidden": false,
"children": {
"category": "tuple",
"id": "line_item",
"label": "Line item",
"hidden": false,
"children": [
{
"width_chars": 10,
"rir_field_names": [
"table_column_code"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "item_code",
"label": "Item SKU",
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [
"table_column_description"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "item_description",
"label": "Description",
"type": "string"
},
{
"width_chars": 10,
"rir_field_names": [
"table_column_quantity"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "item_quantity",
"label": "Quantity",
"type": "number",
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"table_column_amount_base"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "item_amount_base",
"label": "Unit price without VAT",
"hidden": true,
"type": "number",
"can_export": false,
"format": "# ##0.#"
},
{
"width_chars": 10,
"rir_field_names": [
"table_column_amount_total"
],
"constraints": {
"required": false
},
"default_value": null,
"category": "datapoint",
"id": "item_amount_total",
"label": "Total amount",
"type": "number",
"format": "# ##0.#"
}
],
"rir_field_names": []
},
"min_occurrences": null,
"max_occurrences": null,
"default_value": null,
"show_grid_by_default": false,
"rir_field_names": null
},
{
"category": "datapoint",
"id": "master_data_match_po",
"label": "Match data against PO",
"type": "button"
}
],
"icon": null
}
]
Testing function input
If you would be testing the function in the Rossum's UI, please use the input below and do not forget to:
- Fill your token in "rossum_authorization_token". You can get your token over the API - https://api.elis.rossum.ai/docs/#login
- Update the queue ID in "queue" - update it to the queue ID where you uploaded the master data.
{
"request_id": "ae7bc8dd-73bd-489b-a3d2-f5214b209591",
"timestamp": "2020-01-01T00:00:00.000000Z",
"rossum_authorization_token": "...your Rossum auth token...",
"hook": "https://example.rossum.app/api/v1/hooks/781",
"action": "user_update",
"event": "annotation_content",
"annotation": {
"document": "https://example.rossum.app/api/v1/documents/3217006",
"id": 3213337,
"queue": "https://example.rossum.app/api/v1/queues/<... your queue ID ...>",
"schema": "https://example.rossum.app/api/v1/schemas/779827",
"relations": [
"https://example.rossum.app/api/v1/relations/8961"
],
"pages": [
"https://example.rossum.app/api/v1/pages/7658027"
],
"modifier": "https://example.rossum.app/api/v1/users/26026",
"modified_at": "2020-10-12T07:49:05.082613Z",
"confirmed_at": "None",
"exported_at": "None",
"assigned_at": "2020-10-12T07:49:05.082613Z",
"status": "reviewing",
"rir_poll_id": "519a6937a410453181de53a7",
"messages": [],
"url": "https://example.rossum.app/api/v1/annotations/3213337",
"content": [
{
"id": 332573380,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573380",
"children": [
{
"id": 332573381,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573381",
"content": {
"value": "tax_invoice",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "tax_invoice",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": 0.9699772596359253,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "invoice_type",
"validation_sources": [
"human"
],
"time_spent": 5.4
},
{
"id": 332573382,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573382",
"content": {
"value": "eng",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "eng",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": 0.983479380607605,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "language",
"validation_sources": [
"score"
],
"time_spent": 0
},
{
"id": 332573383,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573383",
"content": {
"value": "1",
"normalized_value": "None",
"page": 1,
"position": [
331,
414,
461,
450
],
"rir_text": "143453775",
"rir_page": 1,
"rir_position": [
331,
414,
461,
450
],
"rir_confidence": 0.9907606840133667,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "po_number",
"validation_sources": [
"score"
],
"time_spent": 0
},
{
"id": 332573384,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573384",
"content": {
"value": "52554532",
"normalized_value": "None",
"page": 1,
"position": [
840,
462,
910,
486
],
"rir_text": "52554532",
"rir_page": 1,
"rir_position": [
840,
462,
910,
486
],
"rir_confidence": 0.8872256278991699,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "order_id",
"validation_sources": [
"human"
],
"time_spent": 1.5
},
{
"id": 332573385,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573385",
"content": {
"value": "NO4554352",
"normalized_value": "None",
"page": 1,
"position": [
395,
462,
477,
486
],
"rir_text": "NO4554352",
"rir_page": 1,
"rir_position": [
395,
462,
477,
486
],
"rir_confidence": 0.9115498065948486,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "customer_id",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573386,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573386",
"content": {
"value": "3/1/2019",
"normalized_value": "2019-03-01",
"page": 1,
"position": [
792,
228,
876,
258
],
"rir_text": "3/1/2019",
"rir_page": 1,
"rir_position": [
792,
228,
876,
258
],
"rir_confidence": 0.838154673576355,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "date_issue",
"validation_sources": [
"checks",
"human"
],
"time_spent": 1.1
},
{
"id": 332573387,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573387",
"content": {
"value": "3/31/2019",
"normalized_value": "2019-03-31",
"page": 1,
"position": [
834,
612,
918,
630
],
"rir_text": "3/31/2019",
"rir_page": 1,
"rir_position": [
834,
612,
918,
630
],
"rir_confidence": 0.975411057472229,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "date_due",
"validation_sources": [
"checks",
"score",
"human"
],
"time_spent": 24.8
},
{
"id": 332573388,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573388",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "date_uzp",
"validation_sources": [],
"time_spent": 0
}
],
"category": "section",
"schema_id": "invoice_details_section"
},
{
"id": 332573389,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573389",
"children": [
{
"id": 332573390,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573390",
"content": {
"value": "150342342340",
"normalized_value": "None",
"page": 1,
"position": [
332,
1290,
426,
1314
],
"rir_text": "150342342340",
"rir_page": 1,
"rir_position": [
332,
1290,
426,
1314
],
"rir_confidence": 0.975179135799408,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "account_num",
"validation_sources": [
"score"
],
"time_spent": 0
},
{
"id": 332573391,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573391",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "bank_num",
"validation_sources": [
"human"
],
"time_spent": 1.2
},
{
"id": 332573392,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573392",
"content": {
"value": "NO6513425245230",
"normalized_value": "None",
"page": 1,
"position": [
323,
1308,
445,
1332
],
"rir_text": "NO6513425245230",
"rir_page": 1,
"rir_position": [
323,
1308,
445,
1332
],
"rir_confidence": 0.991435170173645,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "iban",
"validation_sources": [
"score"
],
"time_spent": 0
},
{
"id": 332573393,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573393",
"content": {
"value": "DNBANOKK",
"normalized_value": "None",
"page": 1,
"position": [
321,
1332,
405,
1356
],
"rir_text": "DNBANOKK",
"rir_page": 1,
"rir_position": [
321,
1332,
405,
1356
],
"rir_confidence": 0.8830254673957825,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "bic",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573394,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573394",
"content": {
"value": "30 dage netto",
"normalized_value": "None",
"page": 1,
"position": [
835,
594,
929,
618
],
"rir_text": "30 dage netto",
"rir_page": 1,
"rir_position": [
835,
594,
929,
618
],
"rir_confidence": 0.8971046805381774,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "terms",
"validation_sources": [
"checks"
],
"time_spent": 0
},
{
"id": 332573395,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573395",
"content": {
"value": "undetermined",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "undetermined",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "payment_state",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573396,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573396",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "const_sym",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573397,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573397",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "var_sym",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573398,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573398",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "spec_sym",
"validation_sources": [],
"time_spent": 0
}
],
"category": "section",
"schema_id": "payment_info_section"
},
{
"id": 332573399,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573399",
"children": [
{
"id": 332573400,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573400",
"content": {
"value": "10,383.05",
"normalized_value": "10383.05",
"page": 1,
"position": [
260,
1164,
328,
1194
],
"rir_text": "10,383.05",
"rir_page": 1,
"rir_position": [
260,
1164,
328,
1194
],
"rir_confidence": 0.7503539323806763,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "amount_total_base",
"validation_sources": [
"checks"
],
"time_spent": 0
},
{
"id": 332573401,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573401",
"content": {
"value": "2,595.76",
"normalized_value": "2595.76",
"page": 1,
"position": [
648,
1164,
712,
1194
],
"rir_text": "2,595.76",
"rir_page": 1,
"rir_position": [
648,
1164,
712,
1194
],
"rir_confidence": 0.8279930353164673,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "amount_total_tax",
"validation_sources": [
"checks"
],
"time_spent": 0
},
{
"id": 332573402,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573402",
"content": {
"value": "12,978.81",
"normalized_value": "12978.81",
"page": 1,
"position": [
953,
1164,
1017,
1194
],
"rir_text": "12,978.81",
"rir_page": 1,
"rir_position": [
953,
1164,
1017,
1194
],
"rir_confidence": 0.8024431467056274,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "amount_due",
"validation_sources": [
"human"
],
"time_spent": 2.8
},
{
"id": 332573403,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573403",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "amount_rounding",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573404,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573404",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "amount_paid",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573405,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573405",
"content": {
"value": "nok",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "nok",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": 0.9901123642921448,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "currency",
"validation_sources": [
"score"
],
"time_spent": 0
},
{
"id": 332573406,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573406",
"children": [
{
"id": 332573407,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573407",
"children": [
{
"id": 332573408,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573408",
"content": {
"value": "25",
"normalized_value": "25",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "tax_detail_rate",
"validation_sources": [
"human"
],
"time_spent": 153.3
},
{
"id": 332573409,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573409",
"content": {
"value": "10 383.05",
"normalized_value": "10383.05",
"page": 1,
"position": [
260,
1164,
328,
1194
],
"rir_text": "10 383.05",
"rir_page": 1,
"rir_position": [
260,
1164,
328,
1194
],
"rir_confidence": 0.5714406967163086,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "tax_detail_base",
"validation_sources": [
"checks"
],
"time_spent": 0
},
{
"id": 332573410,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573410",
"content": {
"value": "2 595.76",
"normalized_value": "2595.76",
"page": 1,
"position": [
647,
1164,
711,
1188
],
"rir_text": "2 595.76",
"rir_page": 1,
"rir_position": [
647,
1164,
711,
1188
],
"rir_confidence": 0.8872181177139282,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "tax_detail_tax",
"validation_sources": [
"checks"
],
"time_spent": 0
},
{
"id": 332573411,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573411",
"content": {
"value": "12 978.81",
"normalized_value": "12978.81",
"page": 1,
"position": [
953,
1164,
1017,
1194
],
"rir_text": "12 978.81",
"rir_page": 1,
"rir_position": [
953,
1164,
1017,
1194
],
"rir_confidence": 0.7185622453689575,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "tax_detail_total",
"validation_sources": [
"checks"
],
"time_spent": 0
}
],
"category": "tuple",
"schema_id": "tax_detail"
}
],
"category": "multivalue",
"schema_id": "tax_details",
"grid": "None"
}
],
"category": "section",
"schema_id": "totals_section"
},
{
"id": 332573412,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573412",
"children": [
{
"id": 332573413,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573413",
"content": {
"value": "Convenistra Distribution",
"normalized_value": "None",
"page": 1,
"position": [
389,
1374,
533,
1404
],
"rir_text": "Convenistra Distribution",
"rir_page": 1,
"rir_position": [
389,
1374,
533,
1404
],
"rir_confidence": 0.8720519542694092,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "sender_name",
"validation_sources": [
"human"
],
"time_spent": 5.4
},
{
"id": 332573414,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573414",
"content": {
"value": "Ibution . Kleverveien 4 - 1120 Vestiby",
"normalized_value": "None",
"page": 1,
"position": [
495,
1380,
699,
1404
],
"rir_text": "Ibution . Kleverveien 4 - 1120 Vestiby",
"rir_page": 1,
"rir_position": [
495,
1380,
699,
1404
],
"rir_confidence": 0.9148804545402527,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "sender_address",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573415,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573415",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "sender_ic",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573416,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573416",
"content": {
"value": "NO9756463241MTA",
"normalized_value": "None",
"page": 1,
"position": [
840,
480,
960,
504
],
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "sender_vat_id",
"validation_sources": [
"human"
],
"time_spent": 37
},
{
"id": 332573417,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573417",
"content": {
"value": "InfoNet Workshop",
"normalized_value": "None",
"page": 1,
"position": [
692.5,
302,
811.5,
320
],
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "recipient_name",
"validation_sources": [
"human"
],
"time_spent": 39.6
},
{
"id": 332573418,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573418",
"content": {
"value": "2423 KONGSVINGER\nNorway",
"normalized_value": "None",
"page": 1,
"position": [
246,
282,
380,
324
],
"rir_text": "2423 KONGSVINGER\nNorway",
"rir_page": 1,
"rir_position": [
246,
282,
380,
324
],
"rir_confidence": 0.6000941731862087,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "recipient_address",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573419,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573419",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "recipient_ic",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332573420,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573420",
"content": {
"value": "NO914324274MTA",
"normalized_value": "None",
"page": 1,
"position": [
395,
480,
515.5,
499
],
"rir_text": "NO9143242741",
"rir_page": 1,
"rir_position": [
395,
480,
487,
504
],
"rir_confidence": 0.8956565260887146,
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "recipient_vat_id",
"validation_sources": [
"human"
],
"time_spent": 9.5
}
],
"category": "section",
"schema_id": "vendor_section"
},
{
"id": 332573421,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573421",
"children": [
{
"id": 332573422,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573422",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "notes",
"validation_sources": [],
"time_spent": 0
}
],
"category": "section",
"schema_id": "other_section"
},
{
"id": 332573423,
"url": "https:/example.rossum.app/api/v1/annotations/3213337/content/332573423",
"children": [
{
"id": 332573424,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332573424",
"children": [
{
"id": 332584064,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584064",
"children": [
{
"id": 332584067,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584067",
"content": {
"value": "34432441",
"normalized_value": "None",
"page": 1,
"position": [
252,
677.5,
332.5,
745.5
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_code",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584068,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584068",
"content": {
"value": "HPI Battery 4C 40WHr 2,8AH LI LA098241\n42341-000 Factory SealedH5 Code\nOrigin CN",
"normalized_value": "None",
"page": 1,
"position": [
332.5,
677.5,
647.5,
745.5
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_description",
"validation_sources": [
"human"
],
"time_spent": 211.5
},
{
"id": 332584069,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584069",
"content": {
"value": "3",
"normalized_value": "3",
"page": 1,
"position": [
725.5,
677.5,
781.5,
745.5
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_quantity",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584070,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584070",
"content": {
"value": "StK",
"normalized_value": "None",
"page": 1,
"position": [
781.5,
677.5,
842.5,
745.5
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_uom",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584071,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584071",
"content": {
"value": "645.53",
"normalized_value": "6450000.53",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount_base",
"validation_sources": [
"human"
],
"time_spent": 56.9
},
{
"id": 332584072,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584072",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_rate",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584073,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584073",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_tax",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584074,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584074",
"content": {
"value": "806.91",
"normalized_value": "806.91",
"page": 1,
"position": [
842.5,
677.5,
947.5,
745.5
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount",
"validation_sources": [
"human"
],
"time_spent": 26.2
},
{
"id": 332584075,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584075",
"content": {
"value": "1,936.59",
"normalized_value": "1936.59",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_total_base",
"validation_sources": [
"human"
],
"time_spent": 44.1
},
{
"id": 332584076,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584076",
"content": {
"value": "2420.73",
"normalized_value": "2420.73",
"page": 1,
"position": [
947.5,
677.5,
1037,
745.5
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount_total",
"validation_sources": [
"human"
],
"time_spent": 188.6
},
{
"id": 332584077,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584077",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_other",
"validation_sources": [],
"time_spent": 0
}
],
"category": "tuple",
"schema_id": "line_item"
},
{
"id": 332584065,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584065",
"children": [
{
"id": 332584078,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584078",
"content": {
"value": "62342374",
"normalized_value": "None",
"page": 1,
"position": [
252,
745.5,
332.5,
820
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_code",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584079,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584079",
"content": {
"value": "HPI 11.6-inch HD WLED UWVA touchscreen display\n9023422-001Factory SealedHS Code\nOrigin CN\nSerial Numbers 72624323421",
"normalized_value": "None",
"page": 1,
"position": [
332.5,
745.5,
647.5,
820
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_description",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584080,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584080",
"content": {
"value": "4",
"normalized_value": "4",
"page": 1,
"position": [
725.5,
745.5,
781.5,
820
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_quantity",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584081,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584081",
"content": {
"value": "StK",
"normalized_value": "None",
"page": 1,
"position": [
781.5,
745.5,
842.5,
820
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_uom",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584082,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584082",
"content": {
"value": "2,077.14",
"normalized_value": "2077.14",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount_base",
"validation_sources": [
"human"
],
"time_spent": 43.1
},
{
"id": 332584083,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584083",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_rate",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584084,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584084",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_tax",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584085,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584085",
"content": {
"value": "2596.42",
"normalized_value": "2596.42",
"page": 1,
"position": [
842.5,
745.5,
947.5,
820
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount",
"validation_sources": [
"human"
],
"time_spent": 26
},
{
"id": 332584086,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584086",
"content": {
"value": "8,308.56",
"normalized_value": "8308.56",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_total_base",
"validation_sources": [
"human"
],
"time_spent": 49.1
},
{
"id": 332584087,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584087",
"content": {
"value": "10385.7",
"normalized_value": "10385.7",
"page": 1,
"position": [
947.5,
745.5,
1037,
820
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount_total",
"validation_sources": [
"human"
],
"time_spent": 7.6
},
{
"id": 332584088,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584088",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_other",
"validation_sources": [],
"time_spent": 0
}
],
"category": "tuple",
"schema_id": "line_item"
},
{
"id": 332584066,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584066",
"children": [
{
"id": 332584089,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584089",
"content": {
"value": "999990",
"normalized_value": "None",
"page": 1,
"position": [
252,
820,
332.5,
847
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_code",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584090,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584090",
"content": {
"value": "freight",
"normalized_value": "None",
"page": 1,
"position": [
332.5,
820,
647.5,
847
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_description",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584091,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584091",
"content": {
"value": "1",
"normalized_value": "1",
"page": 1,
"position": [
725.5,
820,
781.5,
847
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_quantity",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584092,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584092",
"content": {
"value": "StK",
"normalized_value": "None",
"page": 1,
"position": [
781.5,
820,
842.5,
847
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_uom",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584093,
"url": "example.rossum.app/api/v1/annotations/3213337/content/332584093",
"content": {
"value": "137.90",
"normalized_value": "137.90",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount_base",
"validation_sources": [
"human"
],
"time_spent": 33.8
},
{
"id": 332584094,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584094",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_rate",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584095,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584095",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_tax",
"validation_sources": [],
"time_spent": 0
},
{
"id": 332584096,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584096",
"content": {
"value": "172.37",
"normalized_value": "172.37",
"page": 1,
"position": [
842.5,
820,
947.5,
847
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount",
"validation_sources": [
"human"
],
"time_spent": 85.8
},
{
"id": 332584097,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584097",
"content": {
"value": "137.90",
"normalized_value": "137.90",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_total_base",
"validation_sources": [
"human"
],
"time_spent": 120.9
},
{
"id": 332584098,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584098",
"content": {
"value": "172.38",
"normalized_value": "172.38",
"page": 1,
"position": [
947.5,
820,
1037,
847
],
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_amount_total",
"validation_sources": [
"human"
],
"time_spent": 57
},
{
"id": 332584099,
"url": "https://example.rossum.app/api/v1/annotations/3213337/content/332584099",
"content": {
"value": "",
"normalized_value": "None",
"page": "None",
"position": "None",
"rir_text": "None",
"rir_page": "None",
"rir_position": "None",
"rir_confidence": "None",
"connector_position": "None",
"connector_text": "None"
},
"category": "datapoint",
"schema_id": "item_other",
"validation_sources": [],
"time_spent": 0
}
],
"category": "tuple",
"schema_id": "line_item"
}
],
"category": "multivalue",
"schema_id": "line_items",
"grid": {
"parts": [
{
"page": 1,
"columns": [
{
"left_position": 252,
"schema_id": "item_code",
"header_texts": [
"Numberi"
]
},
{
"left_position": 332.5,
"schema_id": "item_description",
"header_texts": [
"Description"
]
},
{
"left_position": 647.5,
"schema_id": "item_description",
"header_texts": [
"Description"
]
},
{
"left_position": 725.5,
"schema_id": "item_quantity",
"header_texts": [
"Qty"
]
},
{
"left_position": 781.5,
"schema_id": "item_uom",
"header_texts": [
"Unit"
]
},
{
"left_position": 842.5,
"schema_id": "item_amount",
"header_texts": [
"Unit Price"
]
},
{
"left_position": 947.5,
"schema_id": "item_amount_total",
"header_texts": [
"Amount"
]
}
],
"rows": [
{
"top_position": 677.5,
"type": "data"
},
{
"top_position": 745.5,
"type": "data"
},
{
"top_position": 820,
"type": "data"
}
],
"width": 785,
"height": 169.5
}
]
}
}
],
"category": "section",
"schema_id": "line_items_section"
}
],
"time_spent": 1633,
"metadata": {},
"automated": false
},
"document": {
"id": 314621,
"url": "https://example.rossum.app/api/v1/documents/314621",
"s3_name": "272c2f41ae84a4f19a422cb432a490bb",
"mime_type": "application/pdf",
"arrived_at": "2019-02-06T23:04:00.933658Z",
"original_file_name": "test_invoice_1.pdf",
"content": "https://example.rossum.app/api/v1/documents/314621/content",
"metadata": {}
},
"updated_datapoints": [
332573383
]
}
Updated 4 months ago